Python Booleans
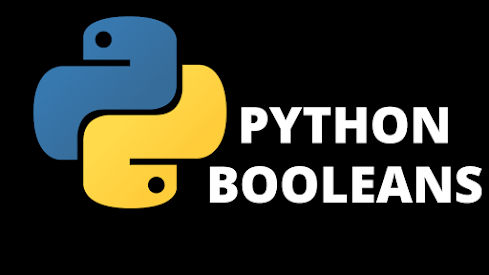
In computer science, the Boolean data type is a data type that has one of two possible values which is intended to represent the two truth values of logic and Boolean algebra. It is named after George Boole, who first defined an algebraic system of logic in the mid 19th century. The boolean data type is either True or False. In Python , boolean variables are defined by the True and False keywords. >>> a = True >>> type(a) <class ' bool '> >>> b = False >>> type(b) <class ' bool '> The output <class ' bool '> indicates the variable is a boolean data type. #Python Boolean #Booleans in Conditionals #Evaluating Values and Variables #False values in Booleans #Class returning False #Boolean Function -Binam Karki